Lesson 2 : Registers
In this lesson you will learn what registers are and what their characteristics are. This is yet another important lesson with things you should know before getting started.
If you know what registers are already, you can skip to lesson 3
What are registers ? |
Most often used registers |
Other registers that you'll use rarely |
More other registers that you'll never use
What are registers
You can't have variables in ASM, so you will need to use the registers.
You could see registers as a kind of variables. But actually they're not.
The registers are storage places where you can store one byte or a word.
Every instruction of the machine language works on a certain register.
For example: When you want to do an addition of two elements in the memory, you would need to copy them to one of the registers first. After which you perform the addition and copy the value back to the memory.
You might wonder why it is not possible to simply add two values from the memory. Well: the answer is simple: memory is slow and registers are very fast.
Some registers are 8 bits (1 byte) wide, others are 16 bits (or 2 bytes) wide. Therefore, The maximum number you can store in a register will be 255 or 65535.
Some 8 bit registers can be used in pairs as 16 bit registers, e.g. HL, DE, BC.
Often-used Registers
Accumulator (A)
The accumulator is 8 bits wide (1 byte). It is the register that has the most possibilities of all registers and is used in almost all the instructions that you give to the processor. When you do math instructions or logical instructions,
the answer will most likely be stored in the accumulator
General purpose registers (B,C,D,E,H,L)
All these registers are 8 bits wide, but they can be used in pairs, so BC, DE and HL are 16 bit registers.
The B register is often used as a counter in loops, HL and DE are mostly used to store addresses or common information
Other Registers (used less)
Stack Pointer (SP)
The Stack Pointer is 16 bits wide and points to the top of the stack. You will learn more about this stack in lesson 12.
Program Counter (PC)
This is a 16 bit register used by the processor to remember the address of the instruction that has to be executed right after the current one is finished. PC is thus increased on each instruction (immediately after the processor copied the current instruction from the memory into an internal register that is not available to the programmer, this updating is also done BEFORE the instruction is executed). The PC is also changed when you jump or make a call to a subroutine. Now think about why the PC is increased BEFORE the instructions are executed and not AFTER.
Flag register
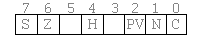
This is a 8 bit register that you won't use in instructions. So why would you need it then?
Well, it contains information on the result of previous instructions. This information is sotred in flags, which are all 1 bit wide and can be in 2 states (true/1 or false/0) according to the results of the last instruction.
Only 6 of the 8 bits are used as flags, the other 2 don't have a any meaning at all.
Not all instructions affect all flags, though. What flags are changed when an instruction is executed, can be found in the opcode table.
Some instructions like EX AF,AF' combine A and F together, this way, you could say that AF is a 16 bit register pair.
For more info about flags, take a look at Lesson 10.
Prime registers (A',F',B',C',D',E',H',L')
I won't tell you much about these registers, because you will use them extremely rarely.
Prime registers can only be used to swap contents with the normal registers A,F,B,C,D,E,H and L. This can be useful when you have to call a subroutine that destroys the contents of all registers.
You swap the contents of the registers first, then you call the function and afterwards, you swap again to get everything back. These registers should never be used when interrupts are enabled (see lesson 20 for more about interrupts) .
Index Registers (IX and IY)
IX and IY are both 16 bits and can be used in the same way as HL, but the instructions are slower and take 1 byte more (to tell the processor that the next instruction works with IX ($DD) or IY ($FD).
These registers can also be used to get info from a table in memory, e.g. ld A,(IX+$05)
The Interrupt register (I)
The interrupt register is 8 bits wide and is used to store the upper byte of the interrupt table. The only 2 instructions that are possible with I are LD A,I and LD I,A.
This is another register that you will use very rarely, you will probably only want to use it for setting up an interrupt. See Lesson 20 for more info on interrupts.
Memory Refresh register (R)
This 8-bit register is actually only of importance when there is dynamic RAM (DRAM). After each instruction, R is increased. This way, it's used as a random number generator by some people, but the random numbers aren't always so random
(same patterns return after a while).
Other Registers
Inside the processor there are even more registers. For example the ALU (Arithmetical and Logical Unit), where the actual calculations are done and registers to temporary store the current instruction.
But since you can't access those registers through instructions, you don't really need to know about them.
Previous lesson |
Contents |
Next lesson
|